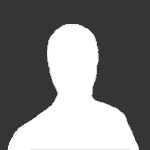
Counting bad parts
Started by
gblack,
8 posts in this topic
Create an account or sign in to comment
You need to be a member in order to leave a comment
Started by
gblack,
You need to be a member in order to leave a comment